#--------------------------------------------------
from VideoCapture import Device
import time, string
import os, sys
import Image
import ImageFilter
import ImageChops
import ImageEnhance
import ImageStat
import ImageGrab
import ImageDraw
import ImageFilter
from masking import*
from dim import*
from draw_mask import* #local python file
cam = Device(devnum=0, showVideoWindow=0)
cam.setResolution(320,240)
masking(interval=input(""))
print "press 'ctrl + c' to terminate"
print 'Duration is measured per seconds'
q = 0
im1 = 0
imsave = 0
upstairs = os.path.join("upstairs")
compose = os.path.join("compose")
DEBUG = 0 #to check intermediate results
Rlim = 255
Blim = 200
Glim = 100 #220
masking()
#-----------------------------------------------------------------------
#call the draw method and add text
draw = ImageDraw.Draw(im)
draw.rectangle(box, outline=(255,0,0))
#for the text location
yoffset = (y - ymin) /2
comment = "moving object"
color = 150,250,0
draw.text((xmax,(ymin-yoffset)), comment, fill=color)
#save results
im.save(compose + '_' + string.zfill(imsave, 1) + '.jpg')
imsave += 1
del draw
function_1
def masking(interval):
interval = input('Enter the amount time between each image capture: ')
quant = interval * .1
starttime = time.time()
while 1:
lasttime = now = int((time.time() - starttime) / interval)
print q
#cam.saveSnapshot(string.zfill(str(i), 2) + '.jpg')
cam.saveSnapshot(upstairs + '_' + string.zfill(q, 1) + '.jpg')
q += 1
#takes each image and opens them incrementally to begin filtering.
im = Image.open(upstairs + '_' + string.zfill(im1, 1) + '.jpg')
im1 += 1
#get a binarized version (threshold auto)
gray = im.convert("L")
bin = gray.convert("1", dither=Image.NONE)
#split into color bands
source = im.split()
R, G, B = 0, 1, 2
#select regions where red is dominant (like the tower in torino)
mask1 = (source[B].point(lambda p: (p > Glim and p) or 0))and (source[R].point(lambda p: (p > Rlim and p) or 0))
mask2 = (source[R].point(lambda p: (p >= Rlim and p) or 0))and (source[B].point(lambda p: (p < Glim and p) or 0))
mask3 = ImageChops.multiply(mask2, bin)
if(DEBUG):
mask3.show()
raw_input()
##filter surrounding
mask4 = mask3.filter(ImageFilter.EDGE_ENHANCE_MORE)
mask5 = mask4.filter(ImageFilter.SMOOTH_MORE)
mask5 = mask5.filter(ImageFilter.SMOOTH_MORE)
#binarize the last mask
mask6 = mask5.convert("1", dither=Image.NONE)
if(DEBUG):
mask6.show()
raw_input()
while now == lasttime:
now = int((time.time() - starttime) / interval)
time.sleep(quant)
function_2
def mask_draw():
mask6 = mask5.convert("1", dither=Image.NONE)
x,y,i,j,k = 0,0,0,0,0
xmin = 250
ymin = 250
xmax = 0
ymax = 0
threshold = 10
#get the size of the image
x,y = mask6.size
#find outer corners of remaining area
for i in range(x):
for j in range(y):
if(mask6.getpixel((i,j))):
if (i < xmin):
xmin = i
if(i > xmax):
xmax = i
if (j < ymin):
ymin = j
if(y > ymax):
ymax = j
#make a box with these boundaries
box = (xmin, ymin, xmax, ymax)
#call the draw method and add text
draw = ImageDraw.Draw(im)
draw.rectangle(box, outline=(255,0,0))
#for the text location
yoffset = (y - ymin) /2
comment = "how we admire the tower of torino"
color = 150,250,0
draw.text((xmax,(ymin-yoffset)), comment, fill=color)
Webcam is required to use this code**
Download code
Object was to track a simple object in real time, with the use of a webcam.
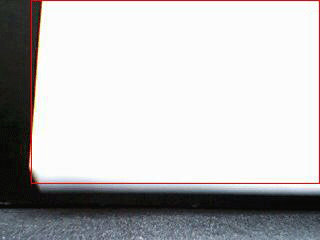
|